4. Week 4 - Embedded programming¶
Day 1 - Introduction to Microcontrollers
“Details about Group Assignment - Types of microcontrollers.”
Day 2 - Using Arduino IDE
Arduino IDE is a user-friendly software application designed specifically for writing, compiling, and uploading code to Arduino boards. It's the go-to tool for anyone starting their journey into electronics and programming with Arduino. Arduino IDE is downloaded and installed to code and communicate with the various microcontrollers.
Setting Up Arduino IDE¶
When starting up Arduino IDE, go to the "File" tab and go to "Preferences" and check the boxes of "compile" and "upload" to allow for the compilation and direct upload.
If the microcontroller is already connected to the PC/laptop, the board will appear in the selection and can be selected directly. If not, you can select the specific type of board through "Select other board and port..." and search up the required board.
For the first time using a new board, some of them might require the installation of their drivers onto the system. This might differ from one device to another but generally you will be following the onscreen prompts.
Basic code examples are available in the Arduino IDE library. To access them, go the "File" tab, then to "Examples", then to "01.Basics", and you can select any basic code from the list. "Blink" is selected here for practice and learning the code. The blink code simply turns on and off the LED on the Arduino board.
In the top left button, the arrow icon is the "Upload" button which will send the code to the board. Using the double backslash will allow to write "Comments" and be colored in gray, where comments do no affect the actual output of the code and is only to make the code more readable for the programmers. The "setup" function is for where the main code is written. The "loop" function is for a code to repeatedly run over and over again.
The delay value is changed to test out the different ways the lights can blink. The first delay function call out is for how long the LED is on while the second one is for how long the LED is off. The value inside is the duration in milliseconds.
Physical Arduino Device¶
The arduino device used is the
Arduino MKR WIFI 1010.
It is connected via a USB cable to the laptop and is setup on the Arduino IDE using the previous sections steps.
Blink - Easy Code - Arduino¶
The challenge here was as follows:
Blink your led but have your blink delay periods be randomized values between 1 second and 5 seconds.
Blink your led but have your blink delay periods be randomized values between 1 second and 5 seconds.
Result:
/*
Blink
Turns an LED on for one second, then off for one second, repeatedly.
Most Arduinos have an on-board LED you can control. On the UNO, MEGA and ZERO
it is attached to digital pin 13, on MKR1000 on pin 6. LED_BUILTIN is set to
the correct LED pin independent of which board is used.
If you want to know what pin the on-board LED is connected to on your Arduino
model, check the Technical Specs of your board at:
https://www.arduino.cc/en/Main/Products
modified 8 May 2014
by Scott Fitzgerald
modified 2 Sep 2016
by Arturo Guadalupi
modified 8 Sep 2016
by Colby Newman
This example code is in the public domain.
https://www.arduino.cc/en/Tutorial/BuiltInExamples/Blink
*/
// the setup function runs once when you press reset or power the board
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(LED_BUILTIN, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
float randOn = random(1,5);
float randOff = random (1,5);
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000*randOn); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(1000*randOff); // wait for a second
}
Blink - Medium Code - Arduino¶
The challenge here was as follows:
Pre code your microcontroller to send a Morse code 1 word message and challenge a friend, family member, your colleagues or your instructor to figure it out. (Refer to the schedule).
Pre code your microcontroller to send a Morse code 1 word message and challenge a friend, family member, your colleagues or your instructor to figure it out. (Refer to the schedule).
Schedule:
Result (2x speed, Morse-Code Word: FOOD):
/*
Blink
Turns an LED on for one second, then off for one second, repeatedly.
Most Arduinos have an on-board LED you can control. On the UNO, MEGA and ZERO
it is attached to digital pin 13, on MKR1000 on pin 6. LED_BUILTIN is set to
the correct LED pin independent of which board is used.
If you want to know what pin the on-board LED is connected to on your Arduino
model, check the Technical Specs of your board at:
https://www.arduino.cc/en/Main/Products
modified 8 May 2014
by Scott Fitzgerald
modified 2 Sep 2016
by Arturo Guadalupi
modified 8 Sep 2016
by Colby Newman
This example code is in the public domain.
https://www.arduino.cc/en/Tutorial/BuiltInExamples/Blink
*/
// the setup function runs once when you press reset or power the board
float LEDON;
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(LED_BUILTIN, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
int dot = 1000;
int dash = 2000;
int gap = 500;
int let = 3000;
int endWord = 5000;
//Letter F
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dot); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dot); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dash); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dot); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(let); // wait for a second
//Letter O
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dash); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dash); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dash); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(let); // wait for a second
//Letter O
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dash); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dash); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dash); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(let);
//Letter D
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dash); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dot); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(gap); // wait for a second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(dot); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(endWord);
}
Blink - Hard Code - Arduino¶
The challenge here was as follows:
You select the duration of the light being on and off while the program is running by entering it in the serial monitor. Write a code that takes the data from the serial monitor and delays by that amount of time.
You select the duration of the light being on and off while the program is running by entering it in the serial monitor. Write a code that takes the data from the serial monitor and delays by that amount of time.
Result (2x Speed; User input 5 first time, 3 second time):
/*
Blink
Turns an LED on for one second, then off for one second, repeatedly.
Most Arduinos have an on-board LED you can control. On the UNO, MEGA and ZERO
it is attached to digital pin 13, on MKR1000 on pin 6. LED_BUILTIN is set to
the correct LED pin independent of which board is used.
If you want to know what pin the on-board LED is connected to on your Arduino
model, check the Technical Specs of your board at:
https://www.arduino.cc/en/Main/Products
modified 8 May 2014
by Scott Fitzgerald
modified 2 Sep 2016
by Arturo Guadalupi
modified 8 Sep 2016
by Colby Newman
This example code is in the public domain.
https://www.arduino.cc/en/Tutorial/BuiltInExamples/Blink
*/
// the setup function runs once when you press reset or power the board
float LEDON;
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(LED_BUILTIN, OUTPUT);
Serial.begin(9600);
}
// the loop function runs over and over again forever
void loop() {
Serial.println("Enter the time value in seconds for the LED to be On: ");
while(Serial.available() == 0){
}
LEDON = Serial.parseFloat();
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000*LEDON); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(1000); // wait for a second
}
Day 3 - Using Thonny (Python IDE)
Using Thonny and Python¶
Thonny is a user-friendly Python Integrated Development Environment (IDE) specifically designed for beginners. It's like a simplified workspace for writing, running, and debugging Python code. In essence, Thonny provides a gentle introduction to programming with Python, making it an excellent choice for those starting their coding journey.
MicroPython is a lean and efficient implementation of the Python 3 programming language designed to run on microcontrollers. These are tiny computers often found in embedded systems like Arduino boards, Raspberry Pi Pico, and many others. A MicroPython device is used as a microcontroller which the programming of MicroPython written in Thonny is implemented on.
For the first time setup of the device, open the Thonny program and go to the "Tools" tab and select "Options..". A pop up window will open. Select the "Interpreter" tab at the top, then select MicroPython (ESP32).
Click on the "Install or update MicroPython (esptool) (UF2)" at the bottom which will pop up a new window with the Target Port found.
Set the MicroPython family to "ESP32", the variant to "Espressif ESP32 / WROOM", and the latest version available. Click on "Install", and the installation procedure will begin and will be completed when a "Done!" prompt is shown at the bottom of the window. Then all the option windows can be closed and the programming can begin.
To be able to view the files saved in the MicroPython device, go to the "View" tab and select "Files". This will add a toolbar on the left of the program where you can view and access the files on the system as well as the MicroPython device.
Once a code has been written, the file can be saved by going to the "File" tab and selecting "Save as...". Select the "MicroPython device" button to allow the code to be run through the microcontroller of the device. Then name the file with a ".py" extension and click on the "OK" button. Finally click on the green button with the white arrow at the top of the screen or the Function 5 "F5" shortcut key to "Run current script" which will run the code.
### Physical MicroPython Device
The MicroPython device used is connected to the laptop via a USB cable. The devices inputs and outputs connects using a telephone wire between the sensor/output device and the MicroPython.
### Blink - Easy Code - Python
### Blink - Medium Code - Python
Result (2x speed, Morse-Code Word: FOOD):
### Blink - Hard Code - Python
### Traffic Light - Python (Thanny)
Result:
The challenge here was as follows:
Blink your led but have your blink delay periods be randomized values between 1 second and 5 seconds.
Result (2x speed):
Blink your led but have your blink delay periods be randomized values between 1 second and 5 seconds.
from machine import Pin
import time
import random
LED = Pin(26,Pin.OUT)
while True:
xrand = random.randint(1,5)
yrand = random.randint(1,5)
LED.value(1)
time.sleep(xrand)
LED.value(0)
time.sleep(yrand)
The challenge here was as follows:
Pre code your microcontroller to send a Morse code 1 word message and challenge a friend, family member, your colleagues or your instructor to figure it out. (Refer to the schedule).
Schedule:
Pre code your microcontroller to send a Morse code 1 word message and challenge a friend, family member, your colleagues or your instructor to figure it out. (Refer to the schedule).
Result (2x speed, Morse-Code Word: FOOD):
from machine import Pin
import time
LED = Pin(26,Pin.OUT)
dot = 1
dash = 2
gap = 0.5
let = 3
endWord = 5
# Letter F
LED.value(1)
time.sleep(dot)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dot)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(let)
# Letter O
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(let)
# Letter O
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(let)
# Letter D
LED.value(1)
time.sleep(dash)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dot)
LED.value(0)
time.sleep(gap)
LED.value(1)
time.sleep(dot)
LED.value(0)
time.sleep(endWord)
The challenge here was as follows:
You select the duration of the light being on and off while the program is running by entering it in the serial monitor. Write a code that takes the data from the serial monitor and delays by that amount of time.
Result (2x Speed; User input 4 for "ON", 2 for "OFF"):
You select the duration of the light being on and off while the program is running by entering it in the serial monitor. Write a code that takes the data from the serial monitor and delays by that amount of time.
from machine import Pin
import time
LED = Pin(26,Pin.OUT)
LEDON = input(float("Enter the On time in seconds: "))
LEDOFF = input(float("Enter the Off time in seconds: "))
while True:
LED.value(1)
time.sleep(LEDON)
LED.value(0)
time.sleep(LEDOFF)
This was just testing and understanding how to use the MicroPython device with another output LED component which resembles a traffic light. The component is connected to the port with three available pins (PIN 17, PIN 18, PIN 19) to be able to manipulate and control each traffic individually.
Result:
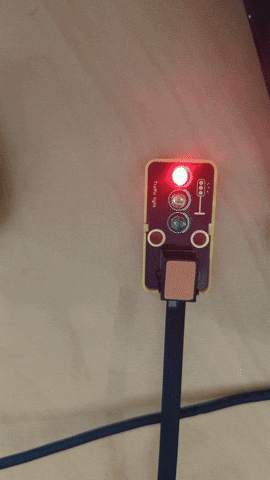
from machine import Pin
import time
RLED = Pin(17,Pin.OUT)
YLED = Pin(18,Pin.OUT)
GLED = Pin(19,Pin.OUT)
RLED.value(0)
YLED.value(0)
GLED.value(0)
while True:
RLED.value(1)
time.sleep(5)
YLED.value(1)
time.sleep(1)
RLED.value(0)
YLED.value(0)
GLED.value(1)
time.sleep(3)
GLED.value(0)
time.sleep(0.5)
GLED.value(1)
time.sleep(0.5)
GLED.value(0)
time.sleep(0.5)
GLED.value(1)
time.sleep(0.5)
GLED.value(0)
time.sleep(0.5)
GLED.value(1)
time.sleep(0.5)
GLED.value(0)
YLED.value(1)
time.sleep(1)
YLED.value(0)
Day 4 - Using Arduino IDE
On this day, documentation was only reviewed and the alternative solutions to the challenges previously mentioned.Personal Notes
- Microcontrollers are easy to use and programme generally and are quite versatile and have many different types.
- Arduino IDE is meant to be easy and quick to learn, but me personally I struggled to understand how the syntax is applied relative to the previous programming languages I already new such as C++ and Matlab.
- Thonny (Python) was a much easier language to comprehend and is very similar to Matlab, making it more enjoyable and less complicated relative to Arduino IDE.
Last update:
July 25, 2024