4. Week 4: Embedded programming¶
This week I learned what microcontrollers are and how to used Arduino IDE and Thonny to programme multiple types of micro-controllers including Ardunio UNO and KidsIOT.
Group Assignment (Microcontrollers)¶

Microcontrollers are integrated circuits that consist of a processor core, memory, and input/output peripherals on a single chip. They are widely used in embedded systems for specific tasks like controlling appliances, automotive systems, and industrial machinery. Microcontrollers have features like timers, communication interfaces, and analog-to-digital converters. Programming involves writing code in languages like C or assembly to define how the microcontroller interacts with peripherals. They are crucial for their compact size, cost-effectiveness, and ability to control diverse systems efficiently.
As a group, we search about different types of microcontrollers using infromation from datasheets. We identified many things such as, the number of pins the microcontroller has, sensors embedded on it and what languages can be used to programme it. Personally, I have contributed to researching about the microcontroller Ardunio Nano 33 IoT. I have used the following data sheet as a reference for my information. All of the group’s findings can be seen in the following link.
Individual Assignment (Arduino)¶

Arduino is an open-source electronics platform based on easy-to-use hardware and software. It allows you to create interactive projects and prototypes. Arduino boards are able to read inputs - light on a sensor, a finger on a button, or a Twitter message - and turn it into an output - activating a motor, turning on an LED, publishing something online.
Setting Up Arduino IDE¶
The Arduino Integrated Development Environment (IDE) is the software used to program Arduino boards. It provides a code editor where you can write your code, a compiler to translate your code into machine language, and tools to upload the code to the board. The Arduino IDE supports the languages C and C++, and it simplifies the process of writing code for Arduino boards, making it accessible for beginners while also offering advanced features for more experienced users. The video below provides an overview of what Arduino is.
To set up the Arduino IDE after installing it, go to file -> preferences, go to the “Show verbose output during” and tick the compile and upload boxes. Enabling verbose output will output more detailed information in the console. This is useful for troubleshooting errors during compilation or upload.


To change where the code files will be saved at, select Sketchbook location -> browse.


When connecting an arduino board, you have to select the port it is connected to in order to be able to send the code to it. When I connected the board I received (Arduino Uno), it was selected automatically. However, if it was not selected automatically, go to “Select other board and port” and search for the name of the board you are using.


Some specific boards will require you to download add-ons to be able to use them with the software. That can be done by going to the board manager and downloading the specific add-on for the board you are using. The board I have used (Arduino Uno) did not require me to download an add-on manually.

Coding with Arduino IDE¶
Ardunio UNO¶

I have used the Arduino UNO as the microcontroller board to test my codes on it. The table below lists some the key features of the microcontroller board. The list of features of the board are available in the group assignment page. The reference page used for the information about the board.
1-Blink Example¶
To practice coding using the Arduino IDE, I have used one of the pre-existing code examples by going to File -> Examples -> 01.Basics -> Blink.

This code basically makes the LED on the Arduino Blink. To adjust the duration the LED stays on and off, I simply changed the numbers values. Note that the duration is in milliseconds.

After changing the code, I have connected the Arduino UNO board to my computer

In the Ardunio IDE, I selected the appropriate port, verified the code and uploaded it to the board.

Code Used:
/*
Blink
Turns an LED on for one second, then off for one second, repeatedly.
Most Arduinos have an on-board LED you can control. On the UNO, MEGA and ZERO
it is attached to digital pin 13, on MKR1000 on pin 6. LED_BUILTIN is set to
the correct LED pin independent of which board is used.
If you want to know what pin the on-board LED is connected to on your Arduino
model, check the Technical Specs of your board at:
https://www.arduino.cc/en/Main/Products
modified 8 May 2014
by Scott Fitzgerald
modified 2 Sep 2016
by Arturo Guadalupi
modified 8 Sep 2016
by Colby Newman
This example code is in the public domain.
https://www.arduino.cc/en/Tutorial/BuiltInExamples/Blink
*/
// the setup function runs once when you press reset or power the board
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(LED_BUILTIN, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for half a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for half a second
}
Click here to download my code
Code output:
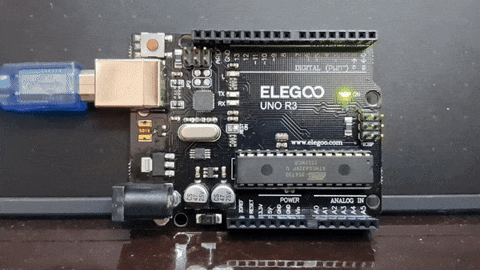
Note: In the videos, I have used a microcontroller board that is identical to the Arduino UNO board but, it is made by a different company called ELEGOO (This is a board that I have at home). This is because the videos I have taken at FabLab with the Arduino UNO were not very clear so, I decided to retake them at home.
After getting a bit used to the Arduino IDE software, we were given 3 challenges to code on our own.
2-Blink Easy¶
Challange: Blink your led but have your blink delay periods be randomized values between 1 second and 5 seconds.
Code used:
/*
Easy mode:
Blink your led but have your blink delay periods be randomized values between 1 second and 5 seconds
*/
void setup() {
pinMode(LED_BUILTIN, OUTPUT); // initialize digital pin LED_BUILTIN as an output.
}
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(random(1000, 5000)); // LED stays on for a random duration between 1 to 5 seconds
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(random(1000, 5000)); // LED stays off for a random duration between 1 to 5 seconds
}
Click here to download my code
Code output:
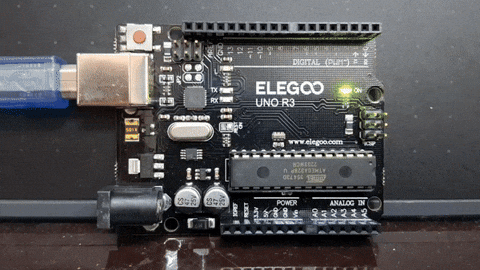
3-Blink Medium¶
Challange: pre code your microcontroller to send a Morse code 1 word message and challenge a friend, family member, your colleagues or your instructor to figure it out.
Code
/*
Medium mode:
pre code your microcontroller to send a Morse code 1 word message and challenge a friend, family member, your colleagues or your instructor to figure it out. (Refer to the schedule)
*/
void setup() {
// put your setup code here, to run once:
pinMode(LED_BUILTIN, OUTPUT);
}
void loop() {
// Spelling Pizza in Morse Code
//P
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(2000); // LED stays on for a 2 seconds (dash)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(2000); // LED stays on for a 2 seconds (dash)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(3000); // LED stays off for 3 seconds (gap between letters)
//I
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(3000); // LED stays off for 3 seconds (gap between letters)
//Z
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(2000); // LED stays on for a 2 seconds (dash)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(2000); // LED stays on for a 2 seconds (dash)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(3000); // LED stays off for 3 seconds (gap between letters)
//Z
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(2000); // LED stays on for a 2 seconds (dash)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(2000); // LED stays on for a 2 seconds (dash)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(3000); // LED stays off for 3 seconds (gap between letters)
//A
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(1000); // LED stays on for a 1 second (dot)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(500); // LED stays off for 0.5 second (gap)
digitalWrite(LED_BUILTIN, HIGH); // turning the LED on
delay(2000); // LED stays on for a 2 seconds (dash)
digitalWrite(LED_BUILTIN, LOW); // turning the LED off
delay(10000); // LED stays off for 3 seconds (morse code finished)
}
Click here to download my code
Code output:
The Morse code spells Pizza.
4-Blink Hard¶
Challange: you select the duration of the light being on and off while the program is running by entering it in the serial monitor. Write a code that takes the data from the serial monitor and delays by that amount of time.
Code used:
/*
Hard mode:
you select the duration of the light being on and off while the program is running by entering it in the serial monitor.
Write a code that takes the data from the serial monitor and delays by that amount of time.
*/
int D; //delay
void setup() {
pinMode(LED_BUILTIN,OUTPUT); // initialize digital pin LED_BUILTIN as an output.
Serial.begin(9600); // Exchanges messages with the serial moniter
D=0;
}
void loop() {
Serial.println("Enter the LED duration"); // Prints the sentence to the serial moniter and creates a new line every time the sentence is written
while (Serial.available() == 0){ //the default value in recived from the serial moniter is 0. this code is used to not do anything while nothing is written in the serial moniter.
}
D = Serial.parseFloat(); //reads the floating value entered in the serial moniter
D=D*1000; // mutiplies the values by 1000 to convert from second to milliseconds
if (D>0){ // when D is greater then 0, light up the LED for the number of seconds writen in the serial moniter
digitalWrite(LED_BUILTIN,HIGH);
delay (D);
digitalWrite(LED_BUILTIN,LOW);
delay(2000);
}
}
Click here to download my code
Code output:
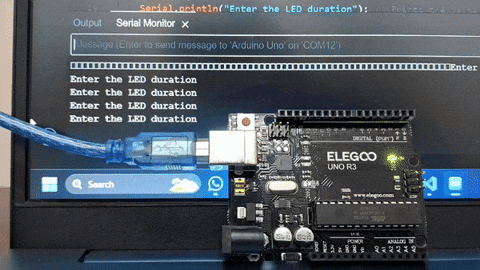
Individual Assignment (Thonny)¶

Thonny is a popular Python integrated development environment (IDE) that is designed for beginners. It provides a simple and clean interface that is easy to use, which makes it ideal for those who are new to programming in Python. Thonny comes with features such as a syntax checker, code completion, and a debugger, which can help users write and debug Python code more easily.
Setting Up Thonny¶
To be able to use Thonny for coding the Kids IoT micro-controller, an interpreter must be downloaded in the micro-controller so that it is able to understand the code that will be uploaded to it. First, connect the micro-controller to the laptop using a USB type C wire.

Then, go to tools -> options.

Go to the interpreter tab and select MicroPython(ESP32).

At the Port and WebREPL tab, select USB Serial @ COM11.

Then, press install or update MicroPython (esptool).

At the MicroPython family tab, select ESP32.

Select Espressif . ESP32 / WROOM as the varient.

Press install.

After the install process is done, press OK.

To be able to see the files on the left side of Thonny, go to the View tab and select files.


Coding with Thonny and KidIOT¶
To code with the KidIOT microcontroller, connect the object you want to it using the one of the ports (for example an LED) and flip the microcontroller to read the pin values. Each port has either 2-3 pin values. Usually, the pin# in the middle the one you should use in the code. However, if it does not work, simply change the pin number in the code to one of the other pins. The pin numbers are written as io## on the bottom of the board.

To start the code, select the green button. To stop or restart the code, select the red button.

1-Blink¶
This code is used to blink an LED.
Code used:
from machine import Pin
import time
LED = Pin(26,Pin.OUT) #initialize digital pin 26 as an output.
while True:
LED.value(1) # Turning the LED on
time.sleep(2) #LED Stays on for 2 seconds
LED.value(0) # Turning the LED off
time.sleep(2) #LED Stays off for 2 seconds
Click here to download my code
Code output:
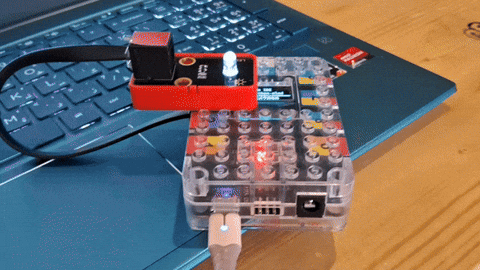
2-Blink Random¶
This code is used to blink an LED for a random duration.
Code used:
from machine import Pin
import time
import random
LED = Pin(26,Pin.OUT)
while True:
LED.value(1) # Turning the LED on
time.sleep(random.randrange(1, 5)) #LED stays on for a random value between 1 to 5 seconds
LED.value(0) # Turning the LED off
time.sleep(random.randrange(1, 5)) #LED stays off for a random value between 1 to 5 seconds
Click here to download my code
Code output:
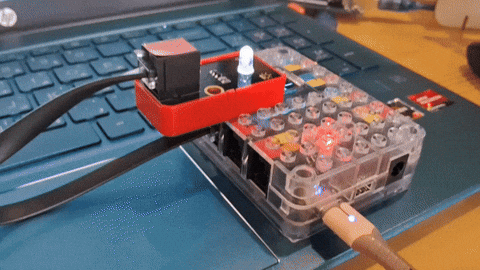
3-Blink Morse Code¶
This code is used to spell a word in Morse code using an LED.
Code used:
from machine import Pin
import time
import random
LED = Pin(26,Pin.OUT) #initialize digital pin 26 as an output
while True:
# S
LED.value(1) #Turning the LED on
time.sleep(1) #LED Stays on for 1 second (dot)
LED.value(0) #Turning the LED off
time.sleep(0.5) #LED Stays of for 0.5 second (gap)
LED.value(1) #Turning the LED on
time.sleep(1) #LED Stays on for 1 second (dot)
LED.value(0) #Turning the LED off
time.sleep(0.5) #LED Stays of for 0.5 second (gap)
LED.value(1) #Turning the LED on
time.sleep(1) #LED Stays on for 1 second (dot)
LED.value(0) #Turning the LED off
time.sleep(3) #LED Stays of for 3 seconds (gap between letters)
#O
LED.value(1) #Turning the LED on
time.sleep(2) #LED Stays on for 2 seconds (dash)
LED.value(0) #Turning the LED off
time.sleep(0.5) #LED Stays of for 0.5 second (gap)
LED.value(1) #Turning the LED on
time.sleep(2) #LED Stays on for 2 seconds (dash)
LED.value(0) #Turning the LED off
time.sleep(0.5) #LED Stays of for 0.5 second (gap)
LED.value(1) #Turning the LED on
time.sleep(2) #LED Stays on for 2 seconds (dash)
LED.value(0) #Turning the LED off
time.sleep(3) #LED Stays of for 3 seconds (gap between letters)
# S
LED.value(1) #Turning the LED on
time.sleep(1) #LED Stays on for 1 second (dot)
LED.value(0) #Turning the LED off
time.sleep(0.5) #LED Stays of for 0.5 second (gap)
LED.value(1) #Turning the LED on
time.sleep(1) #LED Stays on for 1 second (dot)
LED.value(0) #Turning the LED off
time.sleep(0.5) #LED Stays of for 0.5 second (gap)
LED.value(1) #Turning the LED on
time.sleep(1) #LED Stays on for 1 second (dot)
LED.value(0) #Turning the LED off
time.sleep(3) #LED Stays of for 3 seconds (gap between letters)
Click here to download my code
Code output:
4-Powering a Fan and LED¶
This code is used to power a fan and an LED using a button
Code used:
from machine import Pin
from time import sleep
ledred = Pin(12, Pin.OUT) #initialize digital pin 12 as an output (red led).
ledgreen = Pin(13, Pin.OUT) #initialize digital pin 13 as an output (green led).
ledblue = Pin(14, Pin.OUT) #initialize digital pin 14 as an output (blue led).
push_button = Pin(16, Pin.IN) #initialize digital pin 16 as an input (push button).
motor = Pin(23, Pin.OUT) #initialize digital pin 23 as an output (fan motor).
while True:
logic_state = push_button.value()
if logic_state == True: # if the button is push, the light will turn green and the fan motor will turn
ledred.value(1) #Turning the red LED off
ledgreen.value(0) #Turning the green LED on
motor.value(0) #Turning the motor on
else: # if the button is not pushed, the light will turn red and the fan motor will not turn
ledred.value(0) #turning the red led on
motor.value(1) #Turning the motor off
ledgreen.value(1) #turning the green led off
Click here to download my code
Reference code
Code output:
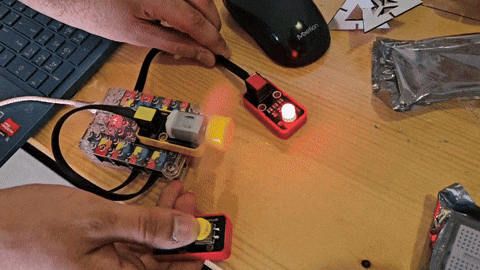