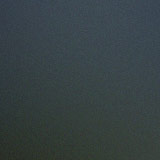
git
Git is a distributed version control system that tracks changes in any set of computer files.
This week I worked on programing a microcontroller. I worked on two different coding languages which are TinkerCAD circuits and Arduino IDE.
"A microcontroller is a compact integrated circuit designed to govern a specific operation in an embedded system. A typical microcontroller includes a processor, memory and input/output (I/O) peripherals on a single chip."
The type I am using is Arduino Nano 33 BLE Sense.
The Arduino Nano 33 BLE looks as seen in the picture below.
But what is Arduino? It is one of the most famous companies dealing with programming and microcontrollers.
As the official Arduino website says:
"Arduino is an open-source electronics platform based on easy-to-use hardware and software. It's intended for anyone making interactive projects."
The Arduino Nano 33 BLE Sense is a hardware device, which is a microcontroller used to make many different projects. It is one of the devices that the official Arduino website recognize as "Enhanced Features". It says that it is the smallest available form from Arduino’s 3.3V AI board.
Arduino Nano 33 BLE Sense has so many features, starting with its sensors:
As seen in the graph, it has five different built-in sensors.
One impressive feature I've read about is that this microcontroller has the possibility of running Edge Computing applications "Artificial Intelligence".
Beside having many useful sensors, the Arduino Nano 33 BLE Sense also comes with Bluetooth so it can be connected to other devices wireless.
And from the name, it has BLE, which stands for Bluetooth Low Energy. It consume low energy, and has low data rate. The video explains how to use BLE.
More features as stated in Arduino Nano 33 BLE Datasheet are Flexible power management, advanced security subsystem, it has 1 MB flash and 256 kB RAM, and others.
Moving to the pinouts on the microcontroller.
digital devices can work when connected to analog pins, while the opposite does not work.
There are different software tools to program the device offline and online like Arduino IDE, Arduino CLI and tinkerCAD. I used Arduino IDE and TinkerCAD to test the difference between coding typically and coding by code blocks.
It seems that every time I upload the program, I need to redo the previous step again which is choosing the correct port.
// the setup function runs once when you press reset or power the board
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(LED_BUILTIN, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(random(1000,5000)); // wait for random time between 1 - 4 seconds
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(3000); // wait for 3 seconds
}
// the setup function runs once when you press reset or power the board
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(LED_BUILTIN, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a 1 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(2000); // wait for 2 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a 1 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(2000); // wait for 2 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a 1 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(2000); // wait for 2 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a 1 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(2000); // wait for 2 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a 1 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(2000); // wait for 2 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a 1 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(500); // wait for a 0.5 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(2000); // wait for 2 second
}
See this video of the code running FABLAB in Morse Code:
or from this link
Code Blocks is an easier way for coding, which uses blocks that connect together to make a code, and a live simulation could be seen.
First I opened TinkerCAD Circuits
Then click on Create New Circuit.
Then I wanted to redo the Morse code I did with Arduino IDE.
One good thing that helped me in Code Blocks is the use of the repeat block. I used it for in which I had more than one dot or dash following each others.
I found Arduino IDE easy to learn from the provided examples and the references in their official website.
TinkerCAD Circuits is more of a fun way to code, it provides some useful Math tools and statements that can be used. A great thing is that after downloading the code you can see the comments of what each code statements do. Moreover, the live simulation gives you a pretty good view of what the code does.